Table of Contents
Learn the basics of ChatGPT API in Python and how to use it for your natural language processing needs.
Welcome to our comprehensive guide on using ChatGPT API in Python. In this article, we will demonstrate the capabilities of ChatGPT through the API provided by OpenAI in Python. Through this article, we will try to cover everything you need to know to get started with ChatGPT.
Why Use ChatGPT?
ChatGPT is an extremely powerful tool that can be used to solve almost all critical NLP problems. It has been developed by OpenAI which is a non-profit organization and launched on 30th November 2022.
According to OpenAI, ChatGPT is a sibling of the InstructGPT model which was trained to follow the instructions given through prompts and provide detailed responses for those prompts.
Before ChatGPT, in NLP research, scientists and researchers have mostly involved in solving NLP problems by training independent models for specific tasks. There was no such model that can handle almost all the critical NLP problems.
With the invention of transformers in NLP space, it has been possible to build large models that can be trained on a corpus of huge size. ChatGPT is also trained on a massive corpus of text data, making it one of the most sophisticated language models available today. It is the variant of the GPT-3 family of pre-trained transformers for text generation. On top of GPT-3, ChatGPT is fine-tuned using both supervised learning and reinforcement learning approaches which makes its responses more prompt and human-like.
Here are just a few interesting use cases that can give you an idea of why you might want to consider using ChatGPT in your own projects:
- Generate high-quality text for a variety of purposes, such as content creation, chatbots, and virtual assistants.
- Generate summaries for complex long documents so that they can be understandable to the 2nd-grade student.
- Build Question Answering applications that can provide detailed answers with proper explanations.
- Generate natural language explanations of complex Programs so that any piece of code can be made understandable to anyone.
- Translate one language to another such as French to English, German, Japanese, etc.
Getting Started with ChatGPT API in Python
In this article, we will demonstrate the Text Completion, Text Summarization, and Question-Answering capabilities of ChatGPT using API available by OpenAI in Python.
To use ChatGPT API in Python, you have to follow the below steps.
Step 1: Register at OpenAI
Create an OpenAI account by registering at https://auth0.openai.com/

Step 2: Generate API Keys
After successfully creating your account in OpenAI, simply log in with your credentials and generate an API key. Next, visit https://platform.openai.com/overview to generate an API key by navigating to the menu “Personal” -> “View API keys” as shown in the below screenshot.

Once you open View API keys you have to create a secret key by clicking on create new secret key button as shown below image.
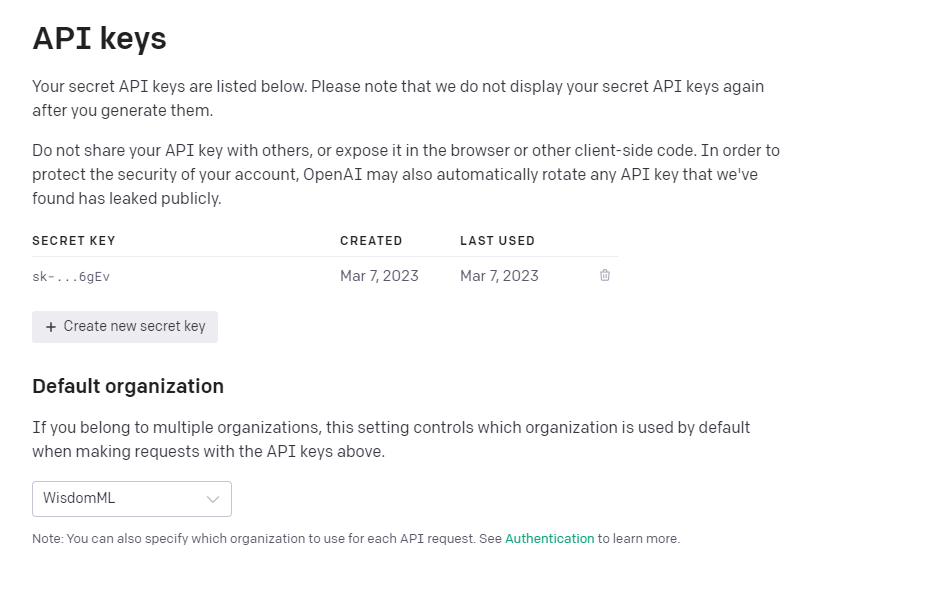
Step 3: Create a ChatGPT Project Folder
The next step is to create a ChatGPT project folder and create a virtual environment where you will be installing all the required libraries for ChatGPT.
mkdir chatgpt-pyproj cd chatgpt-pyproj conda create -n openai python==3.8
Once you have created the project folder and virtual environment, install the required python library.
pip install openai
Step 4: Create Python File
Finally, in this step, we will create a Python file named app.py
in which we will use CHatGPT API for various tasks.
Below is the Python demonstration code for Text Completion which you can also use for your application.
import openai openai.api_key = 'Your Secret Key' def txtcomp(rawtxt): response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "system", "content": "You are a chatbot"}, {"role": "user", "content": rawtxt}, ] ) result = '' for choice in response.choices: result += choice.message.content return result if __name__ == '__main__': rawtxt = "Ramesh and Sumit were very" print(txtcomp(rawtxt))
Output:
close friends since childhood. They went to the same school and college and even ended up working for the same company. They often hung out together and shared their life stories with each other. One day, they decided to go on a trip to the mountains to take a break from their busy lives. They had a great time exploring the beauty of nature and indulging in adventure sports. It strengthened their bond even more and they made unforgettable memories on that trip.
As we can see from the above output, based on the prompt which is rawtxt in our code, the response of ChatGPT is very prompt and natural just like humans. In the above code for text completion, we have used gpt-3.5 turbo which is the actual model used in ChatGPT.
Below is the Python demonstration code for Text Summarization.
import openai openai.api_key = 'Your Secret Key' def txtcomp(rawtxt): response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "system", "content": "You are a chatbot"}, {"role": "user", "content": rawtxt}, ] ) result = '' for choice in response.choices: result += choice.message.content return result def summpred(rawtxt): txt_summ = "Summarize this for a second-grade student:\n\n"+rawtxt completion = openai.Completion.create( model="text-davinci-003", prompt= txt_summ , temperature=0.7, max_tokens=64, top_p=1.0, frequency_penalty=0.0, presence_penalty=0.0 ) response = completion.choices[0].text return response if __name__ == '__main__': rawtxt = """ Jupiter is the fifth planet from the Sun and the largest in the Solar System. It is a gas giant with a mass more than two and a half times that of all the other planets in the Solar System combined, and slightly less than one one-thousandth the mass of the Sun. Jupiter is the third brightest natural object in the Earth's night sky after the Moon and Venus, and it has been observed since prehistoric times. It was named after Jupiter, the chief deity of ancient Roman religion. Jupiter is primarily composed of hydrogen, followed by helium, which constitutes a quarter of its mass and a tenth of its volume. The ongoing contraction of Jupiter's interior generates more heat than the planet receives from the Sun. Because of its rapid rotation rate of 1 rotation per 10 hours, the planet's shape is an oblate spheroid: it has a slight but noticeable bulge around the equator. The outer atmosphere is divided into a series of latitudinal bands, with turbulence and storms along their interacting boundaries. A prominent result of this is the Great Red Spot, a giant storm which has been observed since at least 1831. """ print(summpred(rawtxt))
Output:
Jupiter is a huge planet that is the fifth from the Sun. It is very bright in the night sky and can be seen from Earth since ancient times. It is made up mostly of hydrogen and helium and is shaped like a ball. It has lots of storms, and the biggest one is called the Great Red.
For the summarization task, we have used text-davinci-003
which is the most advanced and powerful version of ChatGPT. We’re also setting the temperature to 0.7, which controls the level of randomness in the generated text. The max_tokens
parameter sets the maximum number of tokens that can be generated, while the n
parameter sets the number of responses to generate.
In the below code, we have demonstrated the Question-Answering capability of the ChatGPT.
import openai openai.api_key = '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' def txtcomp(rawtxt): response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "system", "content": "You are a chatbot"}, {"role": "user", "content": rawtxt}, ] ) result = '' for choice in response.choices: result += choice.message.content return result def summpred(rawtxt): txt_summ = "Summarize this for a second-grade student:\n\n"+rawtxt completion = openai.Completion.create( model="text-davinci-003", prompt= txt_summ , temperature=0.7, max_tokens=64, top_p=1.0, frequency_penalty=0.0, presence_penalty=0.0 ) response = completion.choices[0].text return response def questanspred(rawtxt): completion = openai.Completion.create( model="text-davinci-003", prompt=rawtxt, max_tokens=1024, n=1, stop=None, temperature=0.5, ) response = completion.choices[0].text return response if __name__ == '__main__': rawtxt = " Can you tell me the age of Sun and how much distance it is from earth? " print(questanspred(rawtxt))
Output:
The Sun is estimated to be 4.6 billion years old. It is approximately 93 million miles (150 million kilometers) away from Earth.
That’s it! I hope you like this article and now you may also use ChatGPT API in python with ease.