Canny edge detection is a popular image processing technique used to detect edges in an image. It was developed by John Canny in 1986 and is widely used due to its accuracy and robustness.
The Canny edge detection algorithm involves several steps:
- Noise Reduction: The first step involves removing any noise from the image using a Gaussian filter. This helps to smoothen the image and reduce any irregularities that may interfere with edge detection.
- Gradient Calculation: Next, the algorithm calculates the gradient of the image intensity at each pixel. This is done by applying the Sobel operator to the image in the x and y directions, which gives us the intensity gradients in those directions.
- Non-maximum Suppression: After calculating the gradient, the algorithm identifies the pixels that lie on the edges by performing non-maximum suppression. This step involves scanning through the gradient image and identifying the pixels that have the highest gradient magnitude in the direction of the gradient.
- Double Thresholding: The next step is to apply double thresholding to the image. This involves setting two thresholds, a high threshold, and a low threshold. Pixels with gradient values above the high threshold are considered as strong edges, while those below the low threshold are considered as non-edges. Pixels with gradient values between the two thresholds are considered as weak edges.
- Edge Tracking: The final step is to perform edge tracking, which involves connecting weak edges to strong edges. This is done by following the paths of weak edges that connect to strong edges. If a weak edge is not connected to a strong edge, it is discarded.
So, now we will see how we can implement canny edge detection in python. We will implement canny edge detection on the below sample image.
import cv2 import matplotlib.pyplot as plt %matplotlib inline # Read the original image img = cv2.imread('virat-kohli.jpg') plt.imshow(img)
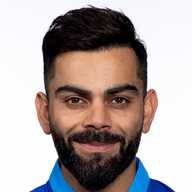
#applying guassian blur img_blur = cv2.GaussianBlur(img, (7, 7), 0) plt.imshow(img_blur)
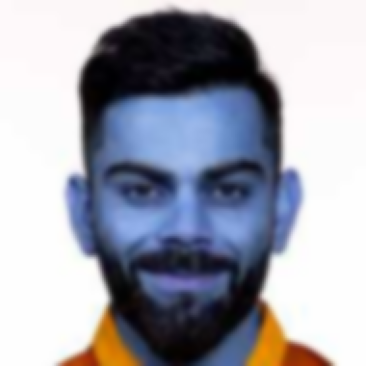
edges = cv2.Canny(image=img_blur, threshold1=80, threshold2=100) plt.imshow(edges)
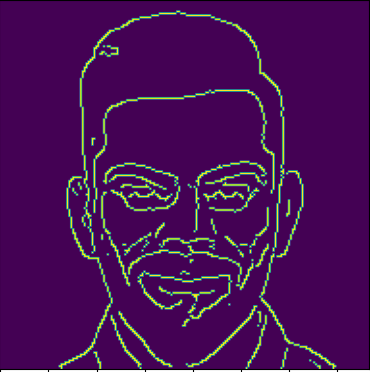
The output of the Canny edge detection algorithm is a binary image where the edge pixels are represented by white pixels and the non-edge pixels are represented by black pixels.
In the above code, we use a lower threshold of 80, and an upper threshold of 100. Based on the parameters, the algorithm has identified the edges accurately in the image, eliminating in the process those less important to the overall structure.
It is to be noted that the results can easily be adjusted by playing with threshold values, so try to experiment with different images, vary the amount of blurring, and try different threshold values to get a feel for things yourself.
In summary, the Canny edge detection algorithm works by identifying the regions of the image with significant changes in intensity, which correspond to edges in the image. The algorithm uses a series of steps to detect these edges, including noise reduction, gradient calculation, non-maximum suppression, double thresholding, and edge tracking.